This is my complete guide to use python firebase Auth in 2021. I used Firebase Auth REST API official documentation to build a simple and clean python codes (api) that allows you to :
- Sign up with email / password.
- Sign in with email / password.
- Send email verification.
- Sign in anonymously.
- Send password reset email.
- Get user data.
- Delete account.
After this article you will be able also to make your own api.
So if you want use firebase authentication in one of your project, You will love today's article.
Let's dive in.
What is firebase authentication
Firebase Authentication provides back-end services, easy-to-use , and ready-made UI libraries to authenticate users to your app . It supports authentication using passwords, phone numbers, popular federated identity providers like Google, Facebook and Twitter, and more.
It is also free !
Create Firebase Project
To create a firebase project :
- Go to https://console.firebase.google.com .
- Click add project.
- Chose name for your project.
- If you want add google analytics you can do it.
- Then click Create project.
Requirements
Project api key
To get started you will need an api key from you firebase project.
Go to your firebase project and click on project settings. and copy the Web API key.
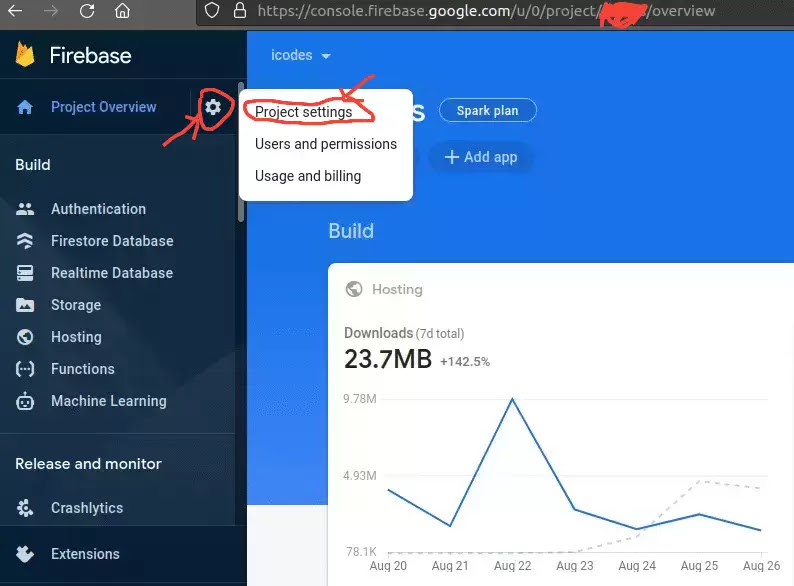
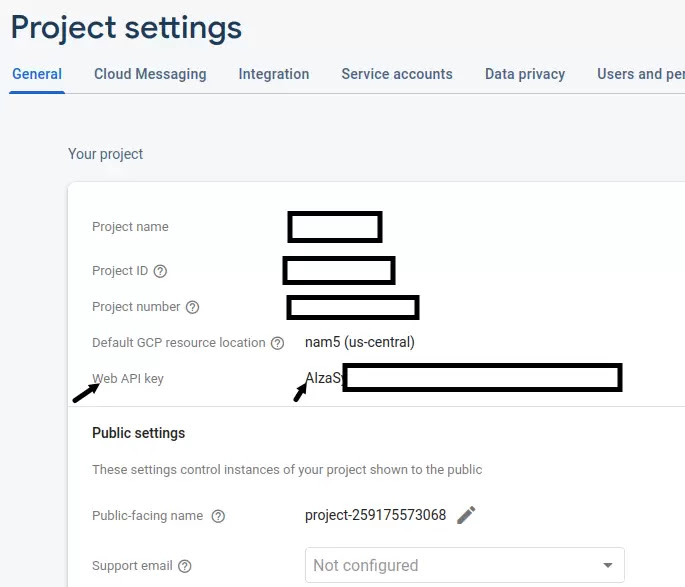
Python requests
You can install requests by:
pip install requests
or
pip3 install requests
check : https://pypi.org/project/requests/ .
How we are going to do this
If you checked the official documentation on each service they give you:
- Request method : We use POST to send data to a server.
- Content-Type : The type of the data we gonna send (in this course we gonna use only JSON ).
- Endpoint : The url we gonna send data to .
- Request Body Payload : The data we gonna send to the server.
- Sample request : An example of a request using curl.
- Sample response : An example of the response from the server.
- Common error codes : The possible errors you can get .
To do all this on python we are going to use requests .
To set the request method to POST
requests.post()
The endpoint is an URL so it's a string.
requests.post('https://identitytoolkit.googleapis.com/v1/accounts:signUp?key={}'.format(apikey))
In the request Body Payload we are going to use a python dict.
details={
'email':email,
'password':password,
'returnSecureToken': True
}
So the sample request will be like this
details={
'email':email,
'password':password,
'returnSecureToken': True
}
# send post request
r=requests.post('https://identitytoolkit.googleapis.com/v1/accounts:signUp?key={}'.format(apikey),data=details)
The
r
contains the response.
To handle the errors we gonna use a function:
def NewUser(email,password):
details={
'email':email,
'password':password,
'returnSecureToken': True
}
# send post request
r=requests.post('https://identitytoolkit.googleapis.com/v1/accounts:signUp?key={}'.format(apikey),data=details)
#check for errors in result
if 'error' in r.json().keys():
return {'status':'error','message':r.json()['error']['message']}
#if the registration succeeded
if 'idToken' in r.json().keys() :
return {'status':'success','idToken':r.json()['idToken']}
And so on ..
Enable Email/Password Sign Up
Allow users to sign up using their email address and password.
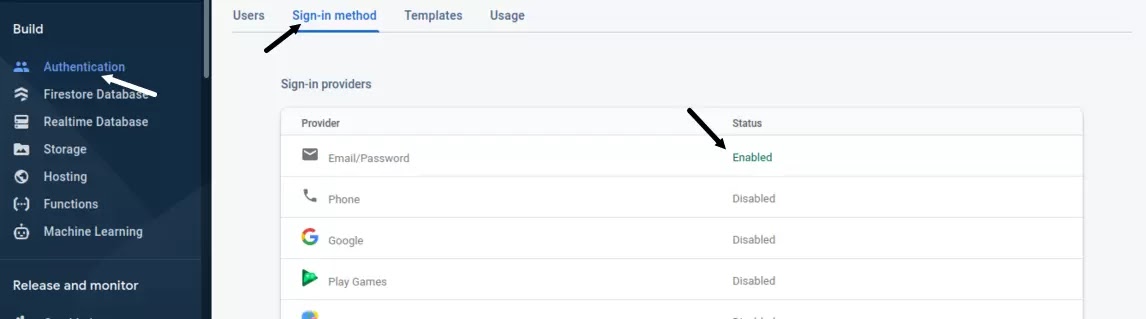
Sign up with email / password
To create a new user account on firebase Auth you need to send a POST request to this URL
https://identitytoolkit.googleapis.com/v1/accounts:signUp
then if the registration succeeded we will get a token to use it later.
Don't panic it's all easy
This is how you do it in python using requests module.
import requests
apikey='.....................'# the web api key
def NewUser(email,password):
details={
'email':email,
'password':password,
'returnSecureToken': True
}
# send post request
r=requests.post('https://identitytoolkit.googleapis.com/v1/accounts:signUp?key={}'.format(apikey),data=details)
#check for errors in result
if 'error' in r.json().keys():
return {'status':'error','message':r.json()['error']['message']}
#if the registration succeeded
if 'idToken' in r.json().keys() :
return {'status':'success','idToken':r.json()['idToken']}
Now this is simple all you have to do is call the function like this :
NewUser('user@example.com','password')# use the email and the password
Output:
You will get something like this if everything is okay :
{'status': 'success', 'idToken': 'eyJhbGciOiJSUzI1NiIsImtpZCI6IjkwMDk1YmM2ZGM2ZDY3NzkxZDdkYTFlZWIxYTU1OWEzZDViMmM0ODYiLCJ0eXAiOiJKV1QifQ.eyJpc3MiOiJodHRwczovL3NlY3VyZXRva2VuLmdvb2dsZS5jb20vaGFja2Vybm9vbi1hcHAiLCJhdWQiOiJoYWNrZXJub29uLWFwcCIsImF1dGhfdGltZSI6MTYzMDA4NTAwOSwidXNlcl9pZCI6Ilk0Q3luTHFVNVRRZGRSSE52MDRzWU54ZWFpdTIiL'}</pre >
or
{'status': 'error', 'message': 'EMAIL_EXISTS'}
it means that the email already registered.
Sign in with email / password
To sign in a registered user on firebase Auth you need to make a POST request to
https://identitytoolkit.googleapis.com/v1/accounts:signInWithPassword</pre >
like this :
import requests
apikey='........................'# the web api key
def SignIn(email,password):
details={
'email':email,
'password':password,
'returnSecureToken': True
}
#Post request
r=requests.post('https://identitytoolkit.googleapis.com/v1/accounts:signInWithPassword?key={}'.format(apikey),data=details)
#check for errors
if 'error' in r.json().keys():
return {'status':'error','message':r.json()['error']['message']}
#success
if 'idToken' in r.json().keys() :
return {'status':'success','idToken':r.json()['idToken']}
Run it :
SignIn('user@example.com','password') #put the actual info
You will get :
{'status': 'success', 'idToken': 'eyJhbGciOiJSUzI1NiIsImtpZCI6IjkwMDk1YmM2ZGM2ZDY3NzkxZDdkYTFlZWIxYTU1OWEzZDViMmM0ODYiLCJ0eXAiOiJKV1QifQ.eyJpc3MiOiJodHRwczovL3NlY3VyZXRva2VuLmdvb2dsZS5jb20vaGFja2Vybm9vbi1hcHAiLCJhdWQiOiJoYWNrZXJub29uLWFwcCIsImF1dGhfdGltZSI6MTYzMDA4NTY0MSwidXNlcl9pZCI6Ilk0Q3luTHFVNVRRZGRSSE'}
or
{'status': 'error', 'message': 'INVALID_PASSWORD'} # Password wrong
{'status': 'error', 'message': 'EMAIL_NOT_FOUND'} # Email not registered</pre >
Send email verification
Now to send an email verification using python. You need to send a POST request to
https://identitytoolkit.googleapis.com/v1/accounts:sendOobCode
You need also the token that you get after sign in or sign up.
import requests
apikey='..................'# the web api key
idToken='eyJh1pVjuYvfX72ewSqUxRNUNoGKgdhsaYdeOjs9OsQ......'#token
def VerifyEmail(idToken):
headers = {
'Content-Type': 'application/json',
}
data='{"requestType":"VERIFY_EMAIL","idToken":"'+idToken+'"}'
r = requests.post('https://identitytoolkit.googleapis.com/v1/accounts:sendOobCode?key={}'.format(apikey), headers=headers, data=data)
if 'error' in r.json().keys():
return {'status':'error','message':r.json()['error']['message']}
if 'email' in r.json().keys():
return {'status':'success','email':r.json()['email']}
run :
VerifyEmail(idToken)
result:
Success
{'status': 'success', 'email': 'user@example.com'}
Failed
{'status': 'error', 'message': 'INVALID_ID_TOKEN'}
The user will receive an email from you like this :
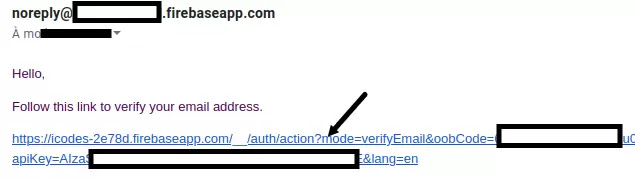
Sign in anonymously
Sometimes you need to use user's features without creating an account. So you can do it on firebase just by sending another POST request to :
https://identitytoolkit.googleapis.com/v1/accounts:signUp
But You have to enable it first

Code :
import requests
apikey='...................'# the web api key
def SignInAnonymously():
headers = {
'Content-Type': 'application/json',
}
data='{"returnSecureToken":"true"}'
r = requests.post('https://identitytoolkit.googleapis.com/v1/accounts:signUp?key={}'.format(apikey), headers=headers, data=data)
if 'error' in r.json().keys():
return {'status':'error','message':r.json()['error']['message']}
if 'idToken' in r.json().keys():
return {'status':'success','idToken':r.json()['idToken']}
Output :
{'status': 'success', 'idToken': 'eyJhbGciOiJSUzI1NiIsImtpZCI6IjkwMDk1YmM2ZGM2ZDY3NzkxZDdkYTFlZWIxYTU1OWEzZDViMmM0'}
Send password reset email
All you need to send an email to reset password using python and the email.Then the user will receive an email to change his password.
just by issuing a POST request to this URL :
https://identitytoolkit.googleapis.com/v1/accounts:sendOobCode
Code :
import requests
apikey='..................'# the web api key
def SendResetEmail(email):
headers = {
'Content-Type': 'application/json',
}
data={"requestType":"PASSWORD_RESET","email":email}
r = requests.post('https://identitytoolkit.googleapis.com/v1/accounts:sendOobCode?key={}'.format(apikey), data=data)
if 'error' in r.json().keys():
return {'status':'error','message':r.json()['error']['message']}
if 'email' in r.json().keys():
return {'status':'success','email':r.json()['email']}
SendResetEmail('user@example.com')
Success :
{'status': 'success', 'email': 'user@example.com'}
if the email isn't registered :
{'status': 'error', 'message': 'EMAIL_NOT_FOUND'}
Get user data
In firebase Auth you can extract user data like :
- is emailVerified
- displayName
- createdAt...
All you need is the Idtoken.
Then send a POST request to
getAccountInfo
endpoint
https://identitytoolkit.googleapis.com/v1/accounts:lookup
Code :
def GetData(idToken):
details={
'idToken':idToken
}
r=requests.post('https://identitytoolkit.googleapis.com/v1/accounts:lookup?key={}'.format(apikey),data=details)
if 'error' in r.json().keys():
return {'status':'error','message':r.json()['error']['message']}
if 'users' in r.json():
return {'status':'success','data':r.json()['users']}
GetData('v7MYMlCA')
Output:
{'status': 'success', 'data': [{'localId': '', 'email': 'user@example.com', 'passwordHash': 'U', 'emailVerified': False, 'passwordUpdatedAt': , 'providerUserInfo': [{'providerId': 'password', 'federatedId': 'user@example.com', 'email': 'user@example.com', 'rawId': 'user@example.com'}], 'validSince': '', 'lastLoginAt': '', 'createdAt': '', 'lastRefreshAt': '2021-08-31T16:37:59.956Z'}]}
Delete account
To delete a user account you need the idToken of the account. Then you send a POST request to :
https://identitytoolkit.googleapis.com/v1/accounts:delete
Code :
def DeleteAccount(idToken):
details={
'idToken':idToken
}
r=requests.post('https://identitytoolkit.googleapis.com/v1/accounts:delete?key={}'.format(apikey),data=details)
if 'error' in r.json().keys():
return {'status':'error','message':r.json()['error']['message']}
return {'status':'success','data':r.json()}
DeleteAccount('jkdhkeguyegyugv')
Output
{'status': 'success', 'data': {'kind': 'identitytoolkit#DeleteAccountResponse'}}
Conclusion
Firebase Auth can be a really useful service and it is also free. so you don't need to deal with the database.Note that you can also connect with Google account,Facebook,github,twitter...
Just by using the rest API you can build other API for more features.